Effective Strategies for Reducing Memory Usage in Python Apps
Written on
Chapter 1: Understanding Memory Optimization
When optimizing the performance of applications, many developers prioritize speed and CPU utilization, often neglecting memory usage—until they encounter out-of-memory issues. Reducing memory consumption is essential for various reasons beyond just preventing crashes.
First and foremost, minimizing memory usage can lead to cost savings, as both CPU and RAM resources have associated expenses. Running inefficient applications wastes memory, so it's prudent to find ways to lessen the memory footprint.
Moreover, large datasets can slow down processing times if they reside on disk rather than in RAM or fast caches. Hence, optimizing memory usage can inadvertently enhance application performance. Additionally, while increasing memory can sometimes boost performance, it’s only effective if there’s available memory on the machine.
Finding Bottlenecks
To effectively cut down memory usage in Python applications, we first need to identify the parts of the code consuming excessive memory. A useful tool for this task is memory_profiler, which allows for line-by-line analysis of memory consumption within specific functions.
To use this tool, install it via pip along with the psutil package to enhance its performance. Decorate the function you intend to analyze with @profile, then execute the profiler using the command python -m memory_profiler. This will provide insights into memory allocation for the designated function.
For a deeper analysis, consider utilizing sys.getsizeof. However, keep in mind that this function may yield inaccurate results for certain data structures. For example, while it accurately measures the size of integers and bytearrays, it only returns the size of the container for more complex structures like lists.
A better alternative is the Pympler library, which offers the asizeof function to accurately report the size of lists and their contents. This tool also tracks class instances and identifies memory leaks, making it a valuable resource for developers.
Optimizing Memory Consumption
Now that we can identify potential memory issues, the next step is to implement solutions. One of the most straightforward methods is to switch to more memory-efficient data structures. Python lists can be quite memory-intensive when storing large arrays of values.
For instance, a simple function can illustrate how creating a list of numbers consumes significant memory—over 350 MiB for 10 million integers. In contrast, using Python’s array module, which stores primitive types, can significantly reduce memory usage to just over 100 MiB.
If mathematical operations are a priority, consider leveraging NumPy arrays, which provide efficient memory use (around 123 MiB) while also offering fast mathematical functions and support for various data types, including complex numbers.
To further optimize memory usage for class-defined objects, utilize the __slots__ class attribute to explicitly declare properties. This action prevents the creation of __dict__ and __weakref__ attributes, thereby saving substantial memory when instantiating multiple objects.
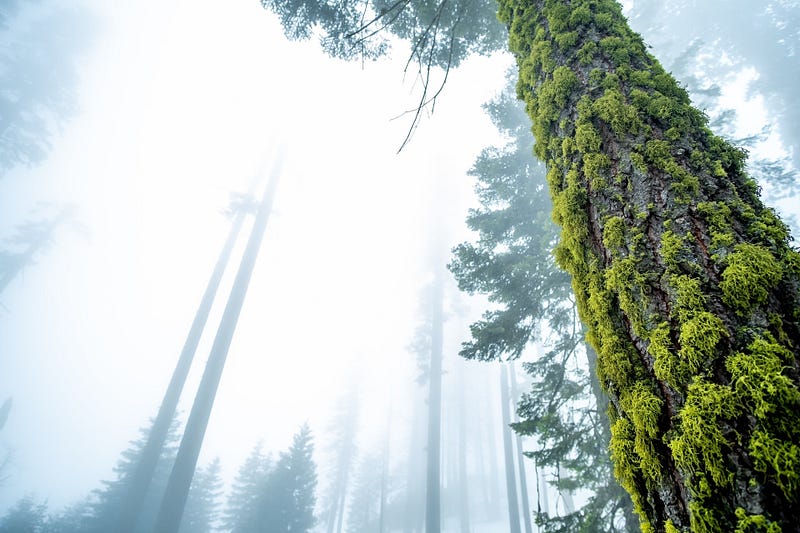
Advanced Memory Management Techniques
To avoid unnecessary memory usage altogether, consider not loading entire datasets at once. Instead, utilize generators that yield elements on demand. This approach can dramatically reduce memory consumption.
Another powerful technique is to use memory-mapped files, which allow for partial data loading from a file. The mmap module in Python’s standard library facilitates the creation of memory-mapped files that function like both files and bytearrays.
To read a memory-mapped file, open it as usual and create a memory-mapped object using the file descriptor. This allows for both file and string operations on the data.
For writing to a memory-mapped file, switch to r+ access mode to enable both reading and writing. Ensure to manage file size adjustments when deleting data, using the move(dest, src, count) method from the mmap module.
Conclusion: Continuous Optimization
Optimizing applications is a complex challenge that varies based on the specific tasks and data types involved. This article has explored common strategies for diagnosing memory issues and implementing effective solutions. However, numerous additional techniques exist for minimizing an application's memory footprint, including employing probabilistic data structures and tree-like structures for efficient string storage.
This article was originally posted at martinheinz.dev