# Automate Your Web Testing with Firefox, Docker, Python, and Selenium
Written on
Chapter 1: Introduction to Automated Web Testing
Are you a developer or QA specialist feeling overwhelmed by the tediousness of manual website testing in Firefox? Do you wish for a streamlined method to automate this process within a Docker container? If so, you’ve come to the right place! In this guide, we will show you how to set up a Docker container that runs Firefox in headless mode for website testing automation, utilizing Python and Selenium. Additionally, we will cover the installation of Xvfb, a virtual display server that allows Firefox to operate smoothly in a headless environment.
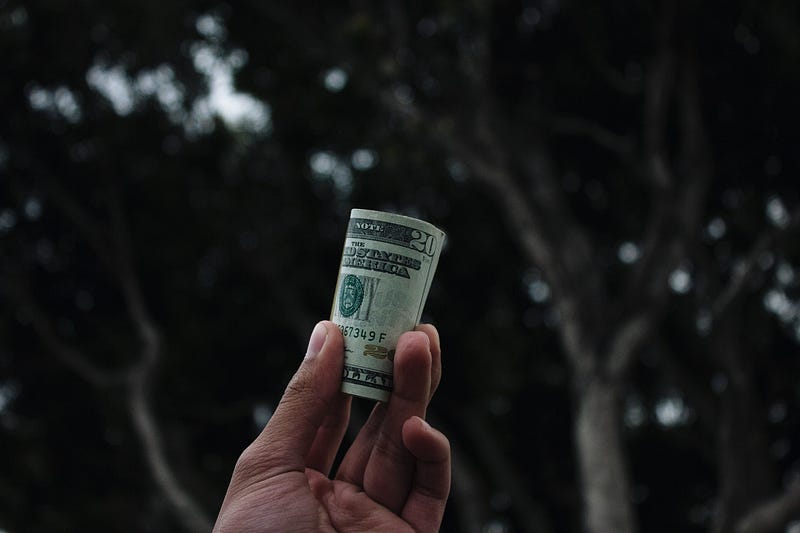
Prerequisites for Automation
Before we begin, ensure that you have the following software ready on your machine:
- Docker
- Python version 3.9 or higher
- Selenium WebDriver
Section 1.1: Creating Your Dockerfile
The initial step involves crafting a Dockerfile that installs Firefox, Xvfb, and the required Python libraries. We will also include the Python script responsible for automating website testing within the Docker container. Below is the Dockerfile:
FROM python:3.9-slim-buster
# Install Firefox and other required packages
RUN apt-get update && apt-get install -y firefox-esr xvfb
# Install necessary Python libraries
RUN pip install selenium
# Install xvfb and xauth
RUN apt-get install -y xvfb xauth
# Include the Python script
COPY main.py .
# Execute the script with xvfb
CMD ["xvfb-run", "--server-args='-screen 0 1024x768x24'", "--auto-servernum", "python", "main.py"]
Section 1.2: Developing the Python Script
Next, we’ll create the main.py Python script. This script will initiate Firefox in headless mode, configure the Firefox binary path, and set up the virtual display server:
from selenium import webdriver
import os
# Enable headless mode
options = webdriver.FirefoxOptions()
options.headless = True
# Define the Firefox binary path
firefox_binary_path = "/usr/bin/firefox-esr"
options.binary_location = firefox_binary_path
# Set the display port as an environment variable
display_port = os.environ.get("DISPLAY_PORT", "99")
display = f":{display_port}"
os.environ["DISPLAY"] = display
# Start the Xvfb server
xvfb_cmd = f"Xvfb {display} -screen 0 1024x768x24 -nolisten tcp &"
os.system(xvfb_cmd)
# Launch the Firefox driver
driver = webdriver.Firefox(options=options)
# Navigate to Google.com
driver.get(url)
# Output the page source
print(driver.page_source)
# Terminate the browser
driver.quit()
Chapter 2: Building and Executing the Docker Container
With both the Dockerfile and Python script prepared, it’s time to build and run the Docker container:
sudo docker build -t my-firefox .
sudo docker run --rm -e DISPLAY=:99 my-firefox
Congratulations! You’ve successfully automated website testing in Firefox using Python, Selenium, and Docker. By running Firefox in headless mode and configuring the virtual display server, you can now execute your tests in a headless setting. This method is scalable and portable, potentially revolutionizing your web testing automation efforts.
Check out the complete project on GitHub: Dockerized-Firefox-for-Web-Scraping
Section 2.1: Conclusion
In this tutorial, we delved into the automation of website testing in Firefox utilizing Python, Selenium, and Docker. By establishing a Docker container and taking advantage of headless mode, we crafted a scalable and portable solution for web testing automation. This approach can be adapted for numerous applications, including web scraping and load testing.
The opportunities don’t stop here! You can broaden your automation toolkit by incorporating additional tools such as:
- Testing frameworks like PyTest or unittest for extensive test scenarios
- Continuous Integration (CI) platforms like Jenkins or GitLab CI to streamline the entire testing workflow
- Other headless browsers such as Chrome or Edge to enhance browser diversity
Feel free to adjust and refine the provided code to meet your specific testing requirements. With this formidable combination of technologies at your disposal, you can significantly enhance the efficiency of your web testing processes and deliver more resilient applications.
If you found this guide beneficial, please give it a thumbs up and share it with your peers. Thank you for reading, and happy testing!